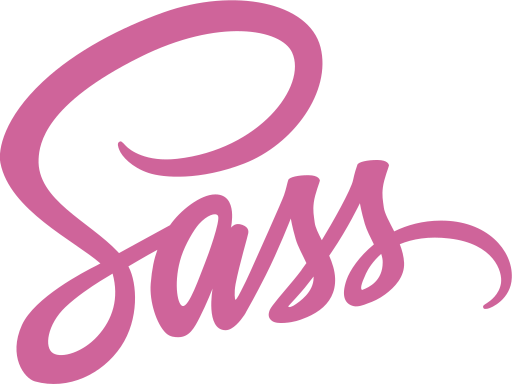
Sass (Syntactically Awesome Style Sheets) is a preprocessor scripting language that extends CSS by allowing you to use features like variables, nested rules, mixins, functions, and inheritance. It helps to make CSS more maintainable, reusable, and easier to manage, especially for large-scale projects. Once you write styles using Sass, it is compiled into standard CSS that the browser can understand.
There are two syntaxes for Sass:
- Sass (Indentation-based syntax): Uses indentation and omits curly braces (
{}
) and semicolons (;
). This syntax is often more concise and readable.
// This is the indented syntax
$primary-color: #333
body
color: $primary-color
- SCSS (Sassy CSS): A more CSS-like syntax with curly braces and semicolons. It is the most commonly used syntax today because it’s easier to transition from regular CSS to SCSS.
// This is the SCSS syntax
$primary-color: #333;
body {
color: $primary-color;
}
Why Use Sass?
- Variables: Store reusable values such as colors, fonts, and dimensions.
- Nesting: Allows nesting of CSS selectors in a hierarchical manner, making it easier to read and maintain.
- Partials: Split your CSS into smaller, reusable files and import them into a main stylesheet.
- Mixins: Define reusable pieces of code that can be used throughout the stylesheet.
- Inheritance: Share styles between selectors.
- Functions: Perform operations like calculations or string manipulations directly in Sass.
Basic Features of Sass
1. Variables
Variables in Sass allow you to store values (such as colors, fonts, and dimensions) and reuse them throughout the stylesheet.
$primary-color: #3498db;
$font-family: Arial, sans-serif;
body {
font-family: $font-family;
color: $primary-color;
}
2. Nesting
You can nest your CSS selectors in a hierarchical structure.
nav {
background: #333;
ul {
list-style: none;
li {
display: inline-block;
a {
color: white;
text-decoration: none;
&:hover {
color: orange;
text-decoration: underline;
}
}
}
}
}
// CSS Output Sample
nav {background: #333;}
nav ul{list-style: none;}
nav ul li {display: inline-block;}
nav ul li a{color: white; text-decoration: none;}
nav ul li a:hover{color: orange; text-decoration: underline;}
3. Partials and Import
You can split your styles into smaller, modular files called partials and import them into one main file. A partial file starts with an underscore (_
).
// _buttons.scss (partial file)
.button {
background-color: $primary-color;
padding: 10px;
}
// main.scss
@import 'buttons';
4. Mixins
Mixins allow you to define reusable chunks of styles.
@mixin button-styles {
padding: 10px;
border-radius: 5px;
background-color: $primary-color;
}
.button {
@include button-styles;
}
5. Inheritance
Inheritance allows one selector to inherit properties from another.
.message {
padding: 10px;
border: 1px solid #ccc;
}
.success {
@extend .message;
border-color: green;
}
6. Functions
You can define and use functions to perform operations.
@function calculate-rem($size-px) {
@return $size-px / 16px * 1rem;
}
body {
font-size: calculate-rem(16px);
}
Installing Sass
Sass can be installed and used in various ways depending on your development environment. Below are some common methods for installing Sass:
1. Using Node.js and npm (Recommended)
The easiest and most common method to install Sass is through Node.js, using npm (Node Package Manager).
- Install Node.js:
- Visit Node.js and download the installer for your operating system.
- After installation, verify by running:
node -v
npm -v
- Install Sass via npm:
Once Node.js is installed, you can install Sass by running the following command in your terminal or command prompt:
npm install -g sass
- Verify Sass Installation:
After installation, verify Sass is installed by running:
sass --version
- Using Sass:
You can now compile Sass by running:
sass source/stylesheets/input.scss build/stylesheets/output.css
2. Using a GUI App (Prepros, Koala)
If you prefer not to work with command lines, you can use a GUI app like:
- Prepros: A cross-platform GUI compiler for Sass, Less, and other preprocessors.
- Koala: A simple GUI application that compiles Sass and other preprocessor languages.
3. Using Ruby (Older Method)
Sass was originally a Ruby project, so it can also be installed using Ruby.
- Install Ruby: If you don’t have Ruby installed, download it from Ruby.
- Install Sass: Once Ruby is installed, run:
gem install sass
4. Integrating Sass with Build Tools
If you use modern build tools like Webpack, Gulp, or Grunt, you can integrate Sass directly into your build pipeline.
- For Webpack:
Install the required loaders:
npm install sass-loader sass webpack --save-dev
- For Gulp:
Install thegulp-sass
plugin:
npm install gulp-sass sass --save-dev
Compiling Sass
To compile Sass into CSS, you can run:
sass source.scss output.css
Or, you can watch for file changes and compile automatically:
sass --watch source.scss:output.css
Conclusion
Sass provides powerful features like variables, nesting, mixins, and inheritance that make writing and maintaining CSS easier and more efficient. It’s simple to install and integrate into any development workflow, especially using tools like Node.js or GUI apps.