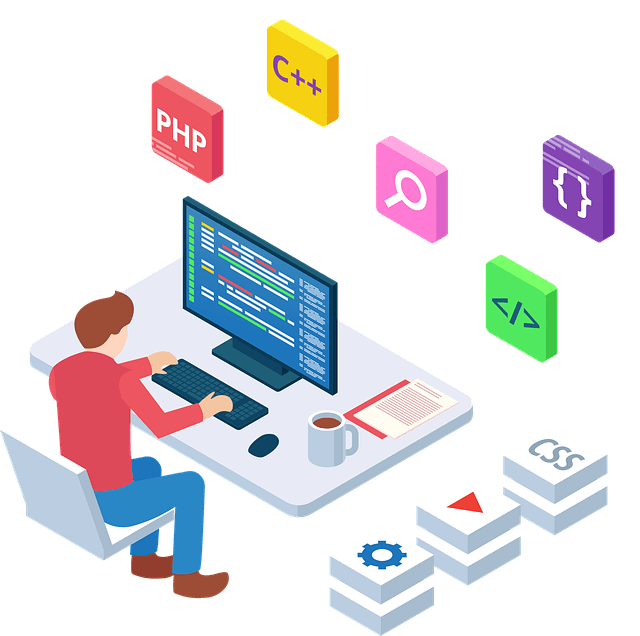
Web development involves creating websites and web applications for the internet or intranets (private networks). It spans a wide range of activities, from building simple static web pages to complex, interactive web applications. Here’s an overview of key concepts, technologies, and practices involved in web development:
1. Types of Web Development
- Frontend Development: Focuses on the client side (what users interact with). It involves creating the layout, design, and user interface (UI) using:
- HTML (HyperText Markup Language): The basic structure of a website.
- CSS (Cascading Style Sheets): Defines the style, layout, colors, fonts, and responsiveness.
- JavaScript: Adds interactivity, dynamic elements, and logic to the website.
- Frontend Frameworks and Libraries:
- React: A JavaScript library for building user interfaces, especially single-page applications (SPA).
- Vue.js: A lightweight framework for building UIs.
- Angular: A complete frontend framework maintained by Google, ideal for large-scale applications.
- Backend Development: Focuses on the server side, where data processing and logic happen. It handles database operations, server communication, and API design. Common backend languages include:
- Node.js: JavaScript runtime for server-side scripting.
- Python (with frameworks like Django, Flask): A powerful language used for web apps with data-intensive needs.
- PHP: Widely used for server-side scripting, particularly with content management systems (CMS) like WordPress.
- Ruby on Rails: Known for its simplicity and developer-friendly syntax.
- Java: Often used for enterprise-level applications.
2. Full-Stack Development
- Full-Stack Developers are skilled in both frontend and backend technologies, allowing them to build and manage both the client-side and server-side of an application. A full-stack development stack might include:
- Frontend: HTML, CSS, JavaScript (React, Vue, Angular)
- Backend: Node.js, Python, PHP, Ruby, etc.
- Databases: MySQL, MongoDB, PostgreSQL, or Firebase for handling data storage.
- APIs: RESTful or GraphQL services for client-server communication.
3. Web Development Workflow
- Planning and Design: Involves creating wireframes, prototypes, and layouts. Tools like Adobe XD, Figma, and Sketch are popular for designing UI/UX.
- Development: The process of writing code and creating the structure, style, and functionality of the website or app.
- Testing: Quality assurance to ensure that the site works across browsers, devices, and is free of bugs.
- Unit Testing: Testing individual components.
- Integration Testing: Ensuring that different parts of the app work together.
- Cross-Browser Testing: Making sure the site works on browsers like Chrome, Firefox, Safari, and Edge.
- Deployment: Moving the code to production servers using services like AWS, Heroku, Netlify, or traditional hosting solutions.
- Maintenance: Continuous updates, bug fixes, and improvements post-launch.
4. Web Development Technologies
- Databases: Storing, retrieving, and managing data.
- SQL Databases: Structured databases like MySQL, PostgreSQL, SQLite.
- NoSQL Databases: Document-based or key-value store databases like MongoDB, Firebase, Redis.
- Version Control: Tracking code changes and collaborating using tools like Git and platforms like GitHub or GitLab.
- APIs:
- RESTful APIs: Follow representational state transfer principles, using HTTP methods like GET, POST, PUT, DELETE.
- GraphQL: A query language for APIs, providing more flexibility in fetching and updating data.
- DevOps: A set of practices that integrates software development and IT operations to automate and streamline deployments.
- CI/CD Pipelines: Continuous Integration/Continuous Deployment tools like Jenkins, Travis CI, and CircleCI.
- Containerization: Using tools like Docker and Kubernetes to manage and deploy applications.
5. Modern Web Development Trends
- Progressive Web Apps (PWAs): Web apps that use modern web capabilities to deliver app-like experiences, including offline functionality and push notifications.
- Single-Page Applications (SPAs): Websites that load a single HTML page and dynamically update content as the user interacts, reducing the need for full-page reloads.
- Serverless Architectures: Backend services managed by cloud providers (e.g., AWS Lambda), allowing developers to focus solely on code.
- Headless CMS: Content management systems (e.g., Strapi, Contentful) that decouple content management from frontend design, allowing for more flexible content delivery across platforms (web, mobile, etc.).
6. Responsive and Mobile-First Design
- With the majority of web traffic coming from mobile devices, creating websites that adapt to different screen sizes is essential. Technologies and practices like media queries in CSS and mobile-first design are key to responsive web development.
7. Web Development Tools
- Code Editors: Tools like Visual Studio Code, Sublime Text, Notepad++, and Atom help developers write and manage code with features like syntax highlighting and debugging.
- Browser Developer Tools: Built into browsers like Chrome and Firefox, these tools help developers inspect code, debug JavaScript, and test performance.
- Package Managers: Tools like npm (Node Package Manager) and Yarn help manage project dependencies and libraries.
8. Security in Web Development
- SSL/TLS: Securing web traffic through HTTPS to protect data transmitted between clients and servers.
- Data Validation: Preventing vulnerabilities like SQL injection or XSS (cross-site scripting) by sanitizing user inputs.
- Authentication & Authorization: Implementing secure login systems using tokens (JWT), OAuth, or multi-factor authentication (MFA).
9. Accessibility
- Ensuring that websites are usable by people with disabilities. This includes proper use of semantic HTML, ARIA roles, keyboard navigation, and screen reader support.
Web development is a dynamic and rapidly evolving field that requires constant learning due to frequent advancements in technology and best practices. Whether you’re working on a small personal blog or a large-scale enterprise web application, the basic principles remain the same, but the tools and techniques evolve continuously.