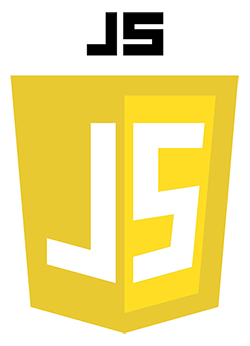
JavaScript is one of the core technologies of web development, alongside HTML and CSS. It is a high-level, interpreted programming language that is primarily used to create interactive and dynamic web content. Here’s a breakdown of the basics of JavaScript:
1. Syntax and Structure
- Case Sensitivity: JavaScript is case-sensitive. For example,
myVariable
andmyvariable
are different. - Statements: Each JavaScript instruction is called a statement, typically ending with a semicolon
;
. - Comments:
- Single-line comments use
//
. - Multi-line comments use
/* ... */
.
- Single-line comments use
2. Variables
Variables are containers for storing data values. In JavaScript, you can declare variables using:
var
: Old way of declaring variables, has function scope.let
: Declares block-scoped variables.const
: Declares block-scoped constants, meaning they cannot be reassigned. Example:
let name = "John"; // String
const age = 25; // Number
3. Data Types
JavaScript is a loosely typed or dynamic language, meaning a variable can hold any type of value:
- Primitive Types:
String
: Textual data, e.g.,"Hello"
.Number
: Numeric data, both integers and floating-point, e.g.,42
or3.14
.Boolean
: Represents logical values,true
orfalse
.Undefined
: A variable that has been declared but not yet assigned a value.Null
: Represents the intentional absence of any object value.Symbol
: Unique and immutable primitive values, introduced in ES6.
let isLoggedIn = true; // Boolean
let greeting = "Hi there!"; // String
let score = 100; // Number
4. Operators
JavaScript includes a wide range of operators to perform operations on variables and values:
- Arithmetic Operators:
+
,-
,*
,/
,%
(modulus) - Assignment Operators:
=
,+=
,-=
,*=
,/=
- Comparison Operators:
==
,===
,!=
,!==
,<
,>
,<=
,>=
- Logical Operators:
&&
(AND),||
(OR),!
(NOT) Example:
let x = 10;
let y = 5;
let sum = x + y; // 15
5. Control Structures
JavaScript provides several ways to control the flow of the program:
- Conditional Statements:
if
,else if
,else
,switch
. - Loops:
for
,while
,do...while
. Example:
let score = 85;
if (score >= 90) {
console.log("Excellent");
} else if (score >= 75) {
console.log("Good");
} else {
console.log("Try Again");
}
6. Functions
Functions are reusable blocks of code that can be executed when called. A function can take parameters and return a value.
Function Declaration:
function greet(name) {
return "Hello, " + name;
}
console.log(greet("Alice")); // Output: "Hello, Alice"
Arrow Functions (ES6):
const add = (a, b) => a + b; console.log(add(3, 4)); // Output: 7
7. Objects
JavaScript is an object-oriented language. Objects are collections of key-value pairs, and they can store multiple values or functions.
Example:
let person = {
firstName: "John",
lastName: "Doe",
age: 30,
greet: function() {
console.log("Hello " + this.firstName);
}
};
console.log(person.firstName); // Output: John
person.greet(); // Output: Hello John
8. Arrays
Arrays in JavaScript are used to store multiple values in a single variable. Arrays are zero-indexed, meaning the first element has an index of 0
.
Example:
let fruits = ["Apple", "Banana", "Cherry"];
console.log(fruits[1]); // Output: Banana
fruits.push("Mango"); // Add a new element
9. Events and DOM Manipulation
JavaScript is widely used to handle user events, such as clicks, and to interact with the Document Object Model (DOM) of a webpage.
Accessing DOM Elements:
let element = document.getElementById("myButton"); element.innerHTML = "Click Me!";
Event Listeners:
document.getElementById("myButton").addEventListener("click", function() { alert("Button clicked!"); });
10. ES6+ Features
Modern JavaScript (ES6 and beyond) introduces several new features, including:
- Let and Const: Block-scoped variables.
- Arrow Functions: Shorter syntax for functions.
- Template Literals: Backticks “ allow for string interpolation.
- Classes: Simplified syntax for object-oriented programming.
- Modules: Ability to export and import code between files. Example of a class:
class Car {
constructor(brand) {
this.brand = brand;
}
drive() {
console.log(this.brand + " is driving");
}
}
let myCar = new Car("Toyota");
myCar.drive(); // Output: Toyota is driving
Conclusion
JavaScript is a versatile language with applications ranging from client-side (in browsers) to server-side (via Node.js). Understanding its basic syntax, control structures, functions, objects, and modern features is key to becoming proficient in web development.
Use this online coding tool for free.